Blur your app preview when backgrounding
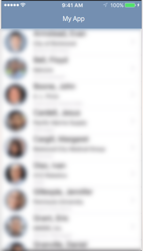
When scrolling through all your open apps on your mobile phone, you get a quick look of what the window will look like when you reactivate the app. For some apps (like banking apps) you don’t want data leaked via the preview image that was taken when the app was backgrounding.
So, here is how you can obscure the preview window when your app is backgrounded.
Blurring the preview on Android
The easiest way to obscure the preview window in Android is by setting two flags in your MainActivity’s OnCreate
public class MainActivity : FormsApplicationActivity
{
protected override void OnCreate(Bundle bundle)
{
base.OnCreate(bundle);
Window.SetFlags(WindowManagerFlags.Secure, WindowManagerFlags.Secure);
}
}
Blurring the preview on iOS
For iOS all the solutions I found didn’t work in all cases. It should be simple, but because I was using Xamarin forms it took me a while to get it working in all cases. I noticed when using a Tabbed layout with a navigation structure, the default blurring mechanism gave some problems. The trick is to find the correct RootViewController before adding the blur. In your AppDelegate add the following methods
private UIVisualEffectView _blurView = null;
public override void OnResignActivation(UIApplication uiApplication)
{
// First find the correct RootViewController
var window = UIApplication.SharedApplication.KeyWindow;
var vc = window.RootViewController;
while (vc.PresentedViewController != null)
{
vc = vc.PresentedViewController;
}
// Add the blur effect
using (var blurEffect = UIBlurEffect.FromStyle(UIBlurEffectStyle.Light))
{
_blurView = new UIVisualEffectView(blurEffect);
_blurView.Frame = UIApplication.SharedApplication.KeyWindow.RootViewController.View.Bounds;
vc.View.AddSubview(_blurView);
}
base.OnResignActivation(uiApplication);
}
public override void OnActivated(UIApplication uiApplication)
{
try
{
if (_blurView != null)
{
_blurView.RemoveFromSuperview();
_blurView.Dispose();
_blurView = null;
}
}
catch { }
base.OnActivated(uiApplication);
}
References
- Find the RootViewController code snippet from Adam Kemp
- Blurring on iOS code snippet from Danny Cabrera